sandy.core.scenegraph.Camera3D
sandy.core.data.Point3D
sandy.primitive.Line3D
sandy.primitive.Torus
- package
- {
- import flash.display.InteractiveObject;
- import flash.display.Sprite;
- import flash.events.Event;
- import flash.events.KeyboardEvent;
- import flash.ui.Keyboard;
- import sandy.core.data.Point3D;
- import sandy.core.Scene3D;
- import sandy.core.scenegraph.Camera3D;
- import sandy.core.scenegraph.Group;
- import sandy.materials.Appearance;
- import sandy.materials.attributes.LightAttributes;
- import sandy.materials.attributes.LineAttributes;
- import sandy.materials.attributes.MaterialAttributes;
- import sandy.materials.ColorMaterial;
- import sandy.materials.Material;
- import sandy.primitive.Line3D;
- import sandy.primitive.Torus;
- public class Example003 extends Sprite
- {
- private var scene:Scene3D;
- private var camera:Camera3D;
- public function Example003()
- {
- camera = new Camera3D(300, 300);
- //camera.x = 100;
- //camera.y = 100;
- camera.z = -400;
- // 使得 camera 物件朝某點拍攝
- //camera.lookAt(0, 0, 0);
- var root:Group = createScene();
- scene = new Scene3D("scene", this, camera, root);
- addEventListener(Event.ENTER_FRAME, enterFrameHandler);
- // 為整個舞台添加鍵盤的 Key Down 事件聆聽器
- stage.addEventListener(KeyboardEvent.KEY_DOWN, keyPressed);
- }
- private function createScene():Group
- {
- var g:Group = new Group();
- // 產生 3DLine 物件, 並設定其參數,分別為:
- // 識別名稱,及 Point3D 物件以定議線條的折點
- var myXLine:Line3D = new Line3D("x-coord", new Point3D( -50, 0, 0),
- new Point3D(50, 0, 0));
- var myYLine:Line3D = new Line3D("y-coord", new Point3D(0, -50, 0),
- new Point3D(0, 50, 0));
- var myZLine:Line3D = new Line3D("z-coord", new Point3D(0, 0, -50),
- new Point3D(0, 0, 50));
- // 產生 Torus 物件,並設定其參數,分別為:
- // 識別名稱、大半徑及小半徑
- var torus:Torus = new Torus("theTorus", 120, 20);
- var materialAttr:MaterialAttributes = new MaterialAttributes(
- new LineAttributes(0.5, 0x2111BB, 0.4),
- new LightAttributes(true, 0.1)
- );
- var material:Material = new ColorMaterial(0xFFCC33, 1, materialAttr);
- material.lightingEnable = true;
- var app:Appearance = new Appearance(material);
- // 將外觀物件套用到顯示物件 tours
- torus.appearance = app;
- torus.rotateX = 30;
- torus.rotateY = 30;
- // 將顯示物件加入舞台顯示群組中
- g.addChild(myXLine);
- g.addChild(myYLine);
- g.addChild(myZLine);
- g.addChild(torus);
- return g;
- }
- private function enterFrameHandler(evt:Event):void
- {
- scene.render();
- }
- private function keyPressed(evt:KeyboardEvent):void
- {
- // 依據使用者所按下的鍵,做不用的處理
- switch(evt.keyCode) {
- case Keyboard.UP:
- // 調整 camera 傾斜的幅度
- camera.tilt += 2;
- break;
- case Keyboard.DOWN:
- camera.tilt -= 2;
- break;
- case Keyboard.RIGHT:
- // 調整 camera 沿Y軸轉向的幅度
- camera.pan -= 2;
- break;
- case Keyboard.LEFT:
- camera.pan += 2;
- break;
- case Keyboard.CONTROL:
- // 調整 camera 沿Z軸卷動的幅度
- camera.roll += 2;
- break;
- case Keyboard.PAGE_DOWN:
- // 調整 camera 鏡頭的遠近幅度
- camera.z -= 5;
- break;
- case Keyboard.PAGE_UP:
- camera.z += 5;
- break;
- }
- }
- }
- }
產出:
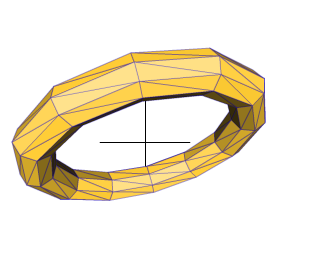
程式碼出處:http://www.flashsandy.org/tutorials/3.0/sandy_cs3_tut03
沒有留言:
張貼留言